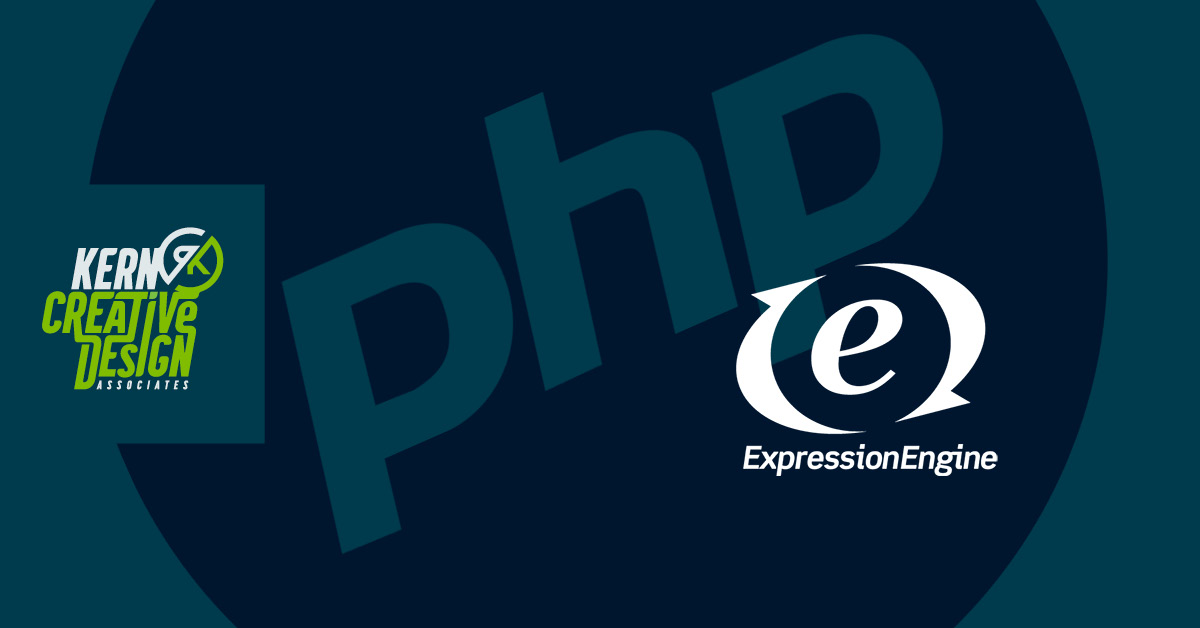
Basic Plugin Development in ExpressionEngine
It is generally not the best idea to include php within your ExpressionEngine templates. EE's plugin architecture allows you so simply write a plugin in minutes in order to have custom code in your pages that are wrapped in ExpressionEngine tags. The docs at https://docs.expressionengine.... do a good job of explaining as well and go into a lot more detail, but I wanted to write this a a basic, practical application.
I think it's important to explain what this post is not. It is not intended to explain (or even give enough information on) how to create plugins of the scope of CartThrob, Calendar, or many others. What it IS though is a post to simply explain how to keep PHP out of your templates. It is also assumed that you can at least write progressive PHP, although the structure of the plugin is object oriented.
The 2 basic File components of a Plugin
2 files are all that are technically needed to write a plugin.
They are placed in their own folder at: system -> users -> addons -> dodads
addon.setup.php
Addon.setup.php is required and is simply the file that will initialize your plugin in the Addons area of the Control Panel. its basic structure is:
<?php return array( 'author' => 'optional - Your name', 'author_url' => 'optional - your website', 'name' => 'DoDads', 'description' => 'Description of what the DoDads plugin does', 'version' => '1.0.0', 'namespace' => 'RussKern\Addons\DoDads' );
pi.dodads.php
This file is your actual plugin. It is a class with its associated methods (functions) in it. You can simply output php generated content with it or pass strings into it to perform varios actions on and then return on the other end.
Below are examples of how to use these basic elements. It is important to note that other things should be done in some of these examples to account for empty parameters and the like, but I want to keep the example clean and easy to follow. The intent is that we can build on the examples below & add other methods to them to keep all our custom code in one place. I personally prefer to keep related methods all in one plugin.
Below is an example of a plugin that requires no information to be passed into it and does one, specific php-related task. It's output will be the viewers IP address.
<?php class DoDads { public function __construct() { } public function users_ip() { // This is a Single Tag method // Use as Single Tag {exp:dodads:users_ip} $ip = $_SERVER["REMOTE_ADDR"]; return $ip; } }
It is really as simple as that.
The intent of most plugins though is to modify existing data or to do things that the Core of EE cannot, and usually they are specific to your application. If you want to pass data into the plugin and modify it, there are 2 ways:
ee()->TMPL->tagdata;
This will take the string of text between a tag pair, for example: {exp:dodads:my_method}{/exp:dodads:my_method} pass it into your method in the plugin, and preform whatever action on it and then you can return it back out.
So in the example below, we know that we have a string that contains a First name and a last name separated by a space. so we will use it as a tag pair and output only the last name. it is used in our template as {exp:dodads:getlastname}Russ Kern{/exp:dodads:getlastname} and it will output "Kern".
<?php class DoDads { public function __construct() { } public function get_lastname() { // This is a Tag Pair Method // Use as tag pair // {exp:dodads:get_last_name}Firstname Lastname{/exp:dodads:get_last_name} $name = ee()->TMPL->tagdata; $parts = explode(' ', $name); $last = parts[1]; return $last; } }
ee()->TMPL->fetch_param('value');
This tag allows you to specify specific parameters within your tag. In the example below, the method "random() will return a random number wit ha minimum and a maximum number of characters. It's use is as a single tag {exp:dodads:random min="" max=""} and it will output a random number with the specified number of characters
<?php class DoDads { public function __construct() { } public function random() { // This is a Single Tag method // Use: {exp:dodads:random min="" max=""} $min = ee()->TMPL->fetch_param('min'); $min = ee()->TMPL->fetch_param('max'); $random = mt_rand(); return $random; } }
And lastly, we can use both methods of passing data into our addon as well, and customize the way the method behaves" In this case we extend the functionality of our "get_last()" method to return a specific part of the name. so the tag {exp:dodads:namesplit half="first"}Russ Kern{/exp:dodads:namesplit} will return "Russ".
<?php class DoDads { public function __construct() { } public function namesplit() { // This is a Tag pair method // Use: {exp:dodads:get_half half=""} //possible values for "part" are first & last $half = ee()->TMPL->fetch_param('part'); $name = ee()->TMPL->tagdata; $parts = explode(' ', $name); switch ($half) { case 'first': $value = parts[0]; break; case 'last': $value = parts[1]; break; default: $value = $name; break; } return $value; } }
I hope that is a helpful, simple way to keep php out of your templates.
Wrapup.
A couple additional notes. I try & comment my addons so I remember how everything works, however, you can add your own Manual by including a Readme.MD file in your addon directory which will be viewable from your addon in the control panel. Also, if you would like to include a custom icon so you can easily see your addon in the sea of addons listed in the CP, ad a 50px x 50px icon.svg file in your addon directory as well.